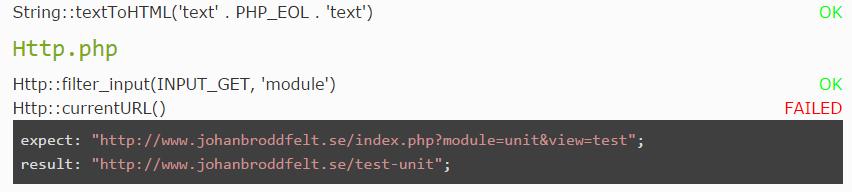
Unit testing the web
In this post I will try to cover some of the pros and cons about unit testing. Show you how I do it and talk about some of the options. I have to admit, I do not spend a lot of time building unit tests, so you should not use this as a resource on how to do unit tests properly. Rather use this post to start thinking about your projects and what need they have in regards to unit testing.
Why testing
Unit tests is not a guarantee that the code delivers what the customer was asking for, it is not a guarantee that your code is free from bugs, it is a way to validate that the code you wrote today did not wreck any of the code you wrote yesterday. This means that you want to have unit tests on code that is used in a lot of situations and you want to create tests before you write the code. But also when you have to fix one of your bugs, make sure you get a test in for the cause of the error before you go and fix the code.
So you say, I'm just writing a small application. My code does not really have any dependencies on other code. Well, next week or next month or even year, you are bound to extend on the code you wrote today. Then you are going to be happy you did that test script.
Do I really need to?
It is a good thing to do, but if your application is heavy on user interfaces and there are not that many layers between the view and the data source and you are working on a web platform that can be easily modified and you have the administrative rights to correct production code on the fly. Then in many cases it is easier to just deploy and let your users test your code. And when it breaks you can just go in and fix it. This is an approach I use in many of my projects and instead of testing I have put a lot of effort into logging and error reports. So that if any error occurs on my website, I get an email describing every detail of what went wrong. But more about logging in another post. If you on the other hand work on an application that need to work and perhaps depend on code installed on different systems that can not be updated as easily, you might want to spend a little bit more time on the testing side of your application.
What options are there?
For testing the interface of a web application the two major tools are Ranorex and Webdriver. I admit I have not yet built a project where I felt that unit tests of the interface was needed. Even though there are some larger projects that I start to feel that it would have been nice to have it. But at least I have created my own php unit test code in order to test some of the functions in my main classes of the project. As you can see below, my code for running tests are simple and still gets the job done.
// classes/Unit.php
OK';
} else {
$validation = str_replace('<', '<', $validation);
$result = str_replace('<', '<', $result);
echo 'FAILED
expect: "' . $validation . '";
result: "' . $result .'";
';
}
echo '
';
}
}
It's only one function that takes a name of the test, the result of the running code and the expected result and if there are any difference then it throws an error. Here is the code I use to implement the tests, and you can see an extract of this page in the top image of this post.
// views/unit/test.php
Unit tests
Db.php
buildFieldString($fields=' * ');
Unit::test('Db::buildFieldString($fields=' * ')', $str, '`id`, `title`, `topic_id`, `summary`, `message`, `embed`, `user_id`, `posted`, `tags`, `publish`, `sent`');
$str = $p->buildFieldString($fields='id, name, mail');
Unit::test('Db::buildFieldString($fields='id, name, mail')', $str, '`id`, `name`, `mail`');
$str = $p->getRealName('my_column_name', '');
Unit::test('Db::getRealName('my_column_name', '')', $str, 'My column name');
$str = $p->strToCamel('my_column_name');
Unit::test('Db::strToCamel('my_column_name')', $str, 'myColumnName');
$str = $p->className('my_table');
Unit::test('Db::className('my_table')', $str, 'MyTable');
?>
String.php
sss');
Unit::test('String::stripDangerousHtml('')', $str, '');
$str = String::stripDangerousHtml('');
Unit::test('String::stripDangerousHtml('')', $str, '');
$str = String::stripDangerousHtml('###a¤¤¤');
Unit::test('String::stripDangerousHtml('###a¤¤¤')', $str, '###a¤¤¤');
$str = String::stripDangerousHtml('Länk');
Unit::test('String::stripDangerousHtml('Länk')', $str, 'Länk');
$str = String::stripDangerousHtml('Länk');
Unit::test('String::stripDangerousHtml('Länk')', $str, 'Länk');
$str = String::stripDangerousHtml('
');
Unit::test('String::stripDangerousHtml('
')', $str, '
');
$str = String::stripDangerousHtml('EM');
Unit::test('String::stripDangerousHtml('EM')', $str, 'EM');
$str = String::stripDangerousHtml('EM');
Unit::test('String::stripDangerousHtml('EM')', $str, 'EM');
$str = String::stripDangerousHtml("'");
Unit::test('String::stripDangerousHtml("'")', $str, "'");
$str = String::fixLinks('text http://www.google.com text');
Unit::test('String::fixLinks("text http://www.google.com text")', $str, 'text http://www.google.com text');
$str = String::fixLinks('text http://www.google.com text');
Unit::test('String::fixLinks("text http://www.google.com text")', $str, 'text http://www.google.com text');
$str = String::textToHTML('text' . PHP_EOL . 'text');
Unit::test('String::textToHTML('text' . PHP_EOL . 'text')', $str, 'text
' . PHP_EOL . 'text');
?>
Http.php
File.php
So to wrap up, unit tests are good to make sure you do not break your code, and that the code actually does what you had in mind. But there are many other kinds of tests that you also should consider that are equally valuable. Here is a list where you can read more about them.
If you want to digg deeper there is also a complete free course on testing at Guru99
- unit test, software